mirror of
https://github.com/langgenius/dify.git
synced 2024-11-16 11:42:29 +08:00
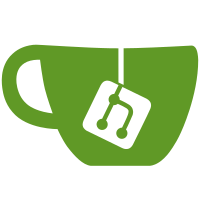
Some checks are pending
Build and Push API & Web / build (api, DIFY_API_IMAGE_NAME, linux/amd64, build-api-amd64) (push) Waiting to run
Build and Push API & Web / build (api, DIFY_API_IMAGE_NAME, linux/arm64, build-api-arm64) (push) Waiting to run
Build and Push API & Web / build (web, DIFY_WEB_IMAGE_NAME, linux/amd64, build-web-amd64) (push) Waiting to run
Build and Push API & Web / build (web, DIFY_WEB_IMAGE_NAME, linux/arm64, build-web-arm64) (push) Waiting to run
Build and Push API & Web / create-manifest (api, DIFY_API_IMAGE_NAME, merge-api-images) (push) Blocked by required conditions
Build and Push API & Web / create-manifest (web, DIFY_WEB_IMAGE_NAME, merge-web-images) (push) Blocked by required conditions
Co-authored-by: crazywoola <427733928@qq.com>
46 lines
1.6 KiB
Python
46 lines
1.6 KiB
Python
import json
|
|
|
|
from core.llm_generator.output_parser.errors import OutputParserError
|
|
|
|
|
|
def parse_json_markdown(json_string: str) -> dict:
|
|
# Get json from the backticks/braces
|
|
json_string = json_string.strip()
|
|
starts = ["```json", "```", "``", "`", "{"]
|
|
ends = ["```", "``", "`", "}"]
|
|
end_index = -1
|
|
for s in starts:
|
|
start_index = json_string.find(s)
|
|
if start_index != -1:
|
|
if json_string[start_index] != "{":
|
|
start_index += len(s)
|
|
break
|
|
if start_index != -1:
|
|
for e in ends:
|
|
end_index = json_string.rfind(e, start_index)
|
|
if end_index != -1:
|
|
if json_string[end_index] == "}":
|
|
end_index += 1
|
|
break
|
|
if start_index != -1 and end_index != -1 and start_index < end_index:
|
|
extracted_content = json_string[start_index:end_index].strip()
|
|
print("content:", extracted_content, start_index, end_index)
|
|
parsed = json.loads(extracted_content)
|
|
else:
|
|
raise Exception("Could not find JSON block in the output.")
|
|
|
|
return parsed
|
|
|
|
|
|
def parse_and_check_json_markdown(text: str, expected_keys: list[str]) -> dict:
|
|
try:
|
|
json_obj = parse_json_markdown(text)
|
|
except json.JSONDecodeError as e:
|
|
raise OutputParserError(f"Got invalid JSON object. Error: {e}")
|
|
for key in expected_keys:
|
|
if key not in json_obj:
|
|
raise OutputParserError(
|
|
f"Got invalid return object. Expected key `{key}` to be present, but got {json_obj}"
|
|
)
|
|
return json_obj
|