mirror of
https://github.com/langgenius/dify.git
synced 2024-11-16 19:59:50 +08:00
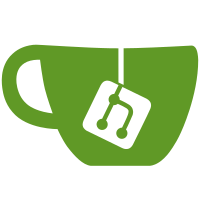
Co-authored-by: Joel <iamjoel007@gmail.com> Co-authored-by: Yeuoly <admin@srmxy.cn> Co-authored-by: JzoNg <jzongcode@gmail.com> Co-authored-by: StyleZhang <jasonapring2015@outlook.com> Co-authored-by: jyong <jyong@dify.ai> Co-authored-by: nite-knite <nkCoding@gmail.com> Co-authored-by: jyong <718720800@qq.com>
60 lines
1.4 KiB
TypeScript
60 lines
1.4 KiB
TypeScript
import { createStore } from 'zustand'
|
|
import type { Features } from './types'
|
|
import { TransferMethod } from '@/types/app'
|
|
|
|
export type FeaturesModal = {
|
|
showFeaturesModal: boolean
|
|
setShowFeaturesModal: (showFeaturesModal: boolean) => void
|
|
}
|
|
|
|
export type FeaturesState = {
|
|
features: Features
|
|
}
|
|
|
|
export type FeaturesAction = {
|
|
setFeatures: (features: Features) => void
|
|
}
|
|
|
|
export type FeatureStoreState = FeaturesState & FeaturesAction & FeaturesModal
|
|
|
|
export type FeaturesStore = ReturnType<typeof createFeaturesStore>
|
|
|
|
export const createFeaturesStore = (initProps?: Partial<FeaturesState>) => {
|
|
const DEFAULT_PROPS: FeaturesState = {
|
|
features: {
|
|
opening: {
|
|
enabled: false,
|
|
},
|
|
suggested: {
|
|
enabled: false,
|
|
},
|
|
text2speech: {
|
|
enabled: false,
|
|
},
|
|
speech2text: {
|
|
enabled: false,
|
|
},
|
|
citation: {
|
|
enabled: false,
|
|
},
|
|
moderation: {
|
|
enabled: false,
|
|
},
|
|
file: {
|
|
image: {
|
|
enabled: false,
|
|
number_limits: 3,
|
|
transfer_methods: [TransferMethod.local_file, TransferMethod.remote_url],
|
|
},
|
|
},
|
|
},
|
|
}
|
|
return createStore<FeatureStoreState>()(set => ({
|
|
...DEFAULT_PROPS,
|
|
...initProps,
|
|
setFeatures: features => set(() => ({ features })),
|
|
showFeaturesModal: false,
|
|
setShowFeaturesModal: showFeaturesModal => set(() => ({ showFeaturesModal })),
|
|
}))
|
|
}
|